Angular for jQuery Professionals – Part 1
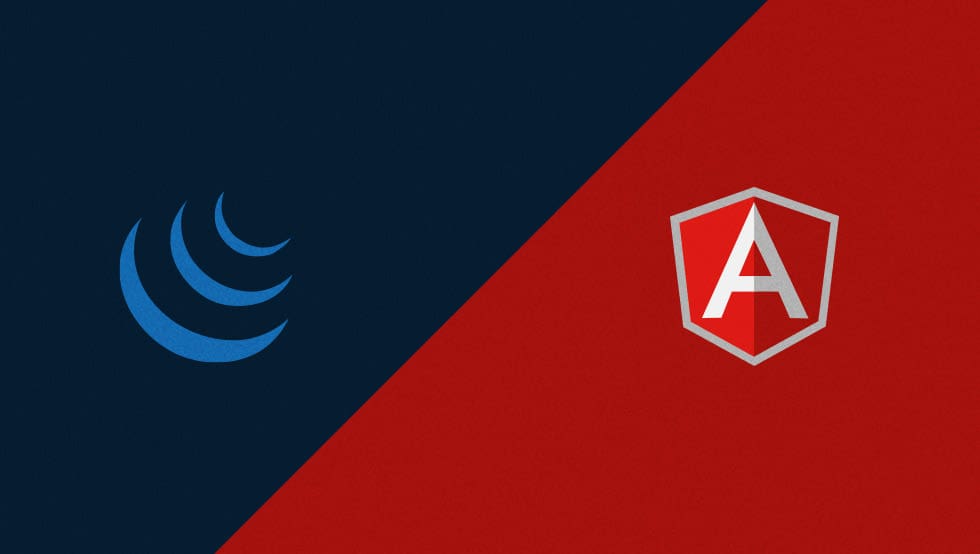
- Clean structure, reusable code and testable components
- One common way to start the angular application development is via NodeJS angular CLI app. This will give ng command to create and maintain the project files
To start a new project
ng new project-name
- The Angular CLI has its own lightweight web server that angular compiles. By default, the port 4200 is used by angular web server.
ng serve
- The root has
node_modules
folder, which stores all the third party libraries. Thesrc
folder has the actual source codes of the project. - The execution starts at main.ts file of the root. The html block that is main.ts will serve is index.html in the root. Package.json file in the root lists out all the dependencies and modules. The typescript compiler setting is in tsconfig.json file there in the root. Typescript compiler converts the typescript to javascript so that web-browsers can understand them.
- The tiny web server of angular CLI uses webpack that automatically bundles all the js and css files. That includes compression and merging to a single file. So unlike the traditional CMS like wordpress which we have to do some extra work for it, here in angular, It does this automatically. If any html of typescript code is changed, the web server automatically detects it,restarts the server and refreshes the browser automatically.
- AngularJS first came in 2010. It was later updated with typescript implementation and more structured and easy for developers. They call it Angular. So the major change is only from version 1 and later. The Angular 2 and later are just minor updates and fixes. To simply term it, AngularJS is for version 1 and Angular simply denotes version 2 and later.
- VSCode is better for Angular applications in terms of auto correction, auto import plugin, shortcuts and syntax highlighting (actually to most of all other javascript frameworks).
TypeScript Fundamentals
Angular uses TypeScript for scripting. Here are some fundamentals of it:
- It’s an extension of javascript. Every script of javascript are a valid typescript. So it can be termed as superset of javascript.
- It has type casting before declaring variables, though not necessarily. Its object oriented i.e it uses classes, constructors, private/public methods etc.
- Typescript transpiler should be installed through npm
npm install typescript
The transpilation can be done by running the command
tsc filname.ts
This creates a .js file in the same folder with same name but as .js extension. When compiling the angular application, this happens automatically under the hood so that browsers can understand.
Things that are in Typescript but not in Javascript
- By default, Typescript generates ES5, which is the oldest version of javascript, supported by all browsers. (later version are ES2016, ES2017…). Let keyword is supported after 2015 version, which may not be supported by some older browser versions.
- Typescript compiler reports error but still tries to generate valid javascript code
- Type Assertions i.e to explicitly tell the compiler the type of variable can be done by two ways
let message;
message = ‘abcd’
let ct = (<string>message);
Or
let ct = (message as string);
- To define a function, it is not necessary to write the word function, instead can use arrow function
let c = function(p){ console.log(p) }
The above function in typescript can be replaced with arrow function as
let c = (p) => { console.log(p) }
This way, the code is cleaner.
- If you are familiar with PHP, the classes, methods and properties usage are exactly the same. Initialize the class with
new
keyword and call the members with. The properties inside class is accessible viathis
keyword. - Declaring constructor function is absolutely similar to PHP. the
constructor()
is reserved function inside the class. Adding ? in constructor parameter make it optional.
class human{ leg: ‘2’; hand: ‘3’ constructor(leg ?: number){ } }
- The access modifiers are private, public and protected. The usage and meaning are exactly the same way.
class human{ private leg: ‘2’; public hand: ‘3’ constructor(leg ?: number){ } } let John = new human();
- The access modifiers can be used directly inside constructor
- To export the class to use outside of the file, use export keyword
export class human{ private leg: ‘2’; public hand: ‘3’ constructor(leg ?: number){ } }
To import
import {CLASS_NAME} from ‘RELATIVE_PATH/MODULE_OR_FILENAME’;
MODULE_OR_FILENAME should be without .ts or without typescript extension.
- Class, method, attributes etc can be exported from a component (a file) and be used in other component (file) like the way stated above.
- Each component has html markup. The custom element is defined and attached inside the component.
- Components can be generated using angular CLI
ng g c hero
import { Component, NgModule, OnInit } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { platformBrowserDynamic } from '@angular/platform-browser-dynamic'; class Hero { id: number; name: string; } @Component({ selector: 'app-root', template: ` <h1>{{title}}</h1> <pre>{{heroes | json}}</pre> `, styleUrls: ['app/app.component.css'] }) @NgModule({ imports: [BrowserModule], declarations: [AppComponent], exports: [AppComponent], bootstrap: [AppComponent] }) export class AppModule {} platformBrowserDynamic().bootstrapModule(AppModule); const HEROES: Hero[] = [ { id: 1, name: 'Bombasto' }, { id: 2, name: 'Tornado' }, { id: 3, name: 'Magneta' } ]; function getHeroes(): Promise<Hero[]> { return Promise.resolve(HEROES); // TODO: get hero data from the server; }
It will then automatically generate all the basic necessary files for you in a separate directory on the current directory. It will also automatically update app.module.ts file, which is the main file to register all the modules in the application
- The beauty of Angular is the data-binding. The dom value of a variable is automatically updated if the variable value is updated. This is done internally by the angular framework. Variables can be displayed in the template by using the two curly braces:
{{ the-variable }}
This curly brace also accepts strings to concatenate with the variable using + operator as in normal javascript concatenation. This even accepts the function call from there that is defined inside the class of the component.
- Backticks can be used in template string (string implementation, not .html template) to make multiline
- The event handling is done by old angular way, eg: for button click
<button (click)=”myFunc()” ></button>
myFunc is the function defined inside the component class for this specific component.
Input field can be bound with # method. Can be passed into the function on click event like this:
<input type=”text” #username />
<button (click)=”myFunc(username.value)” ></button>
This will need to import forms module